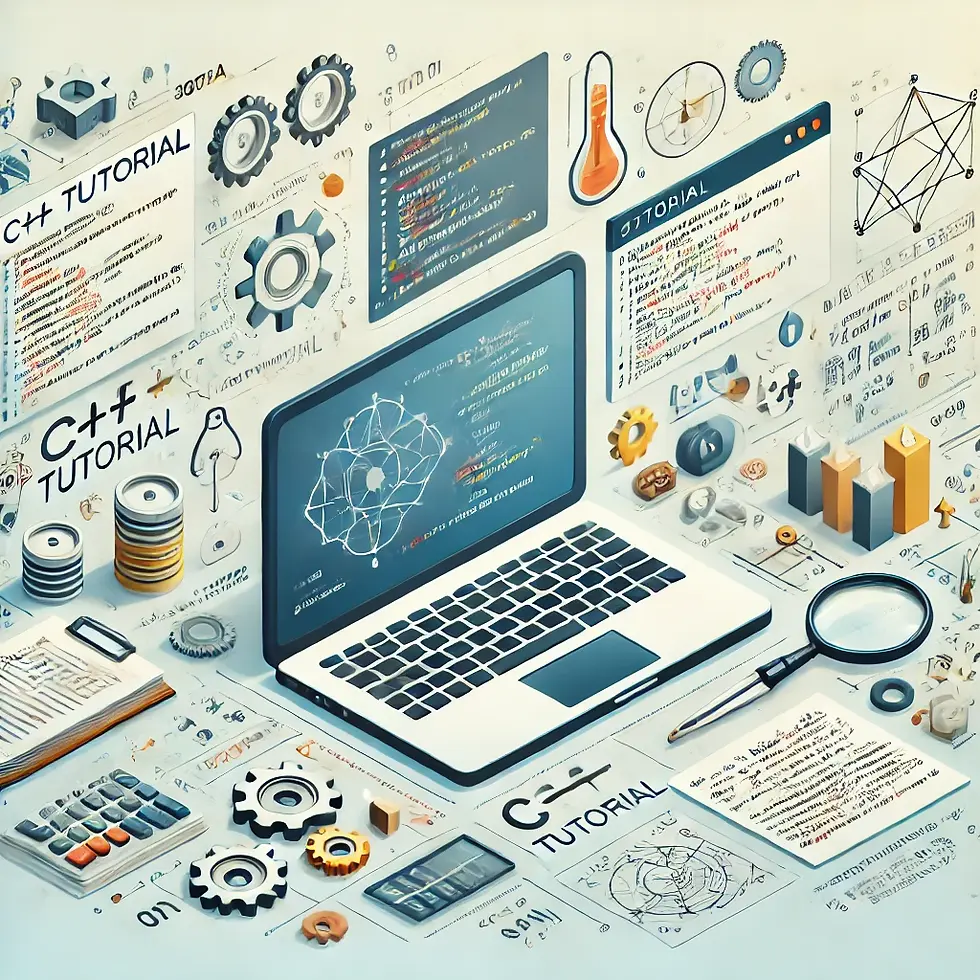
Introduction to Paradigms in Programming Fundamentals
A paradigm in programming is a style or way of programming. Think of it as a method or approach used to solve problems with code. Just like in school, you have different ways to solve math problems (like using algebra or geometry), in programming, there are different paradigms we will learn in the introduction to programming fundamentals
Types of Paradigms:
Object-Oriented Programming (OOP): Think of this like building with Legos. You create small, reusable pieces (objects) and put them together to create something bigger. Java and C++ are examples of OOP languages.
Functional Programming: This is like a factory assembly line. You pass data through a series of functions, each transforming the data. Haskell and parts of Python use functional programming.
Imperative Programming: This is about giving the computer a sequence of tasks to perform. It focuses on how to achieve the result. Languages like C and JavaScript are imperative.
Procedural Programming: This is like following a recipe. You write a sequence of steps (or procedures) to solve a problem. A subset of imperative programming, procedural programming specifically organizes these instructions into procedures or functions. C is a good example of a procedural language.
Generic Programming: This paradigm allows you to write code that works with any data type. It's like writing a formula that works for any number. C++ templates are an example of generic programming.
Structured Programming: This paradigm focuses on improving the clarity, quality, and development time of a software program. It uses constructs like loops, conditionals, and subroutines. Most high-level languages support structured programming.
What is a Compiler, Linker, and Interpreter?
Compiler: A tool that translates code from a high-level programming language (like C++ or Java) into machine language that the computer can understand. Think of it as a translator that translates a book from English to Chinese.
Linker: After the compiler translates the code, the linker takes different pieces of code and combines them into a single executable program. It’s like assembling a puzzle from individual pieces.
Interpreter: Instead of translating the whole program at once, an interpreter translates and executes the code line by line. Python often uses an interpreter.
IDE (Integrated Development Environment)
An IDE is a software application that provides a comprehensive set of tools for software development in a single interface. It typically includes a code editor, compiler, debugger, and other development tools. An IDE helps programmers write, test, and debug their code more efficiently.
Features of an IDE:
Code Editor: A text editor with features like syntax highlighting and code completion.
Compiler/Interpreter Integration: Allows you to compile or interpret your code directly within the IDE.
Debugger: Helps you find and fix errors in your code by allowing you to step through code, set breakpoints, and inspect variables.
What is a Script?
A script is a type of program that is usually written in an interpreted language. Scripts are often used for automating tasks. For example, a Python script might automate data entry.
Regarding C++ code, it is generally not referred to as a script. C++ is a compiled language, meaning that its code is transformed into machine code by a compiler before it can be executed. The compiled machine code is typically an executable file that can be run directly by the operating system.
Types of Programming Languages:
Category | Language Type | Description |
Low-Level Languages | Machine Language | Directly understood by the computer's CPU. |
Assembly Language | Uses symbols and lacks the abstraction of high-level languages. | |
High-Level Languages | Procedural Languages | Like C, focus on a sequence of commands. |
Object-Oriented Languages | Like Java and C++, focus on objects and data manipulation. | |
Scripting Languages | Like Python and JavaScript, often used for automation and web development. |
How C++ Works:
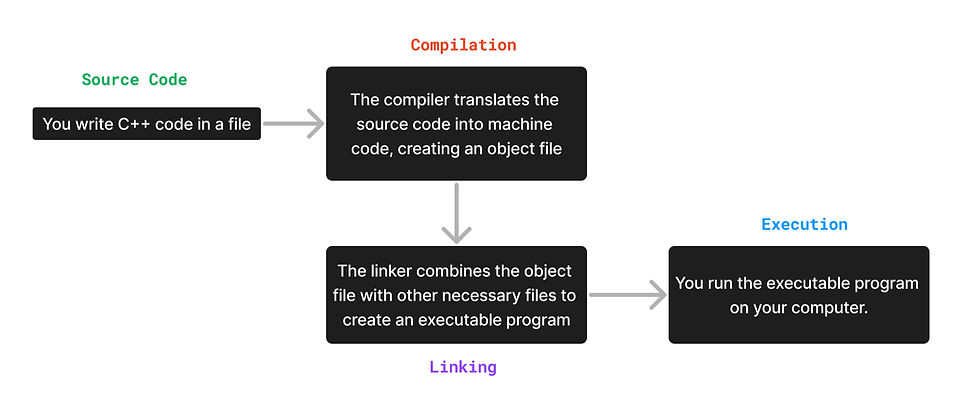
How Java Works:
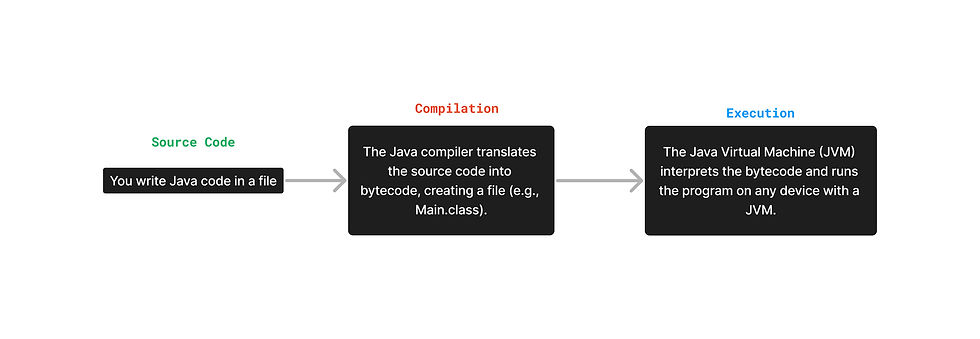
C++ vs Java:
Language | Characteristic | Description |
C++ | Compiled | Code is translated into machine language before running. |
Multiple Paradigms | Supports procedural, object-oriented, generic, and functional programming. | |
Java | Interpreted and Compiled | Code is compiled into bytecode, which runs on the Java Virtual Machine (JVM). |
Object-Oriented | Strongly emphasizes OOP. |
The Java Virtual Machine (JVM) is like a special box that makes your computer understand and run Java programs. When you write a Java program, it gets turned into a language that the JVM understands. The JVM then tells your computer what to do, so your Java program can work on any computer that has a JVM. It's like having a translator that helps your computer understand Java.
Types of Programming Languages Based on Typing:
Strongly Typed Languages: In these languages, each variable has a specific type (like a label), and you have to stick to it. If you make a mistake, the computer catches it early. This makes the code more reliable. Examples are Java and Python.
Weakly Typed Languages: These languages are more flexible with types and let you change them without much fuss. But this can sometimes cause unexpected problems. Examples are JavaScript and PHP.
Statically Typed Languages: In these languages, you must say what type of variable you are using when you write the code. The computer knows the type before running the program. Examples are C++ and Java.
Dynamically Typed Languages: In these languages, you don’t have to declare the type of a variable. The computer figures it out while the program is running, and the type can change as needed. Examples are Python and JavaScript.
Memory
Memory in a computer is like a big storage area where data and programs are kept while they are being used. Think of it as the computer's short-term memory, which helps it remember what it needs to do next.
In simple terms, memory is like a workspace where the computer keeps all the stuff it’s currently working on, making sure everything runs smoothly.
Summary
In this tutorial, we covered key computer science concepts:
Programming Paradigms: Procedural, object-oriented, functional, imperative, generic, and structured programming.
Language Comparison: Differences between C++ and Java in terms of compilation and paradigms.
Compiler, Linker, Interpreter: Roles in transforming and running code.
Scripts: Programs written in interpreted languages for automation.
Types of Languages: Low-level vs. high-level, and strong vs. weak, static vs. dynamic typing.
How C++ and Java Work: Steps from source code to execution.
Comments