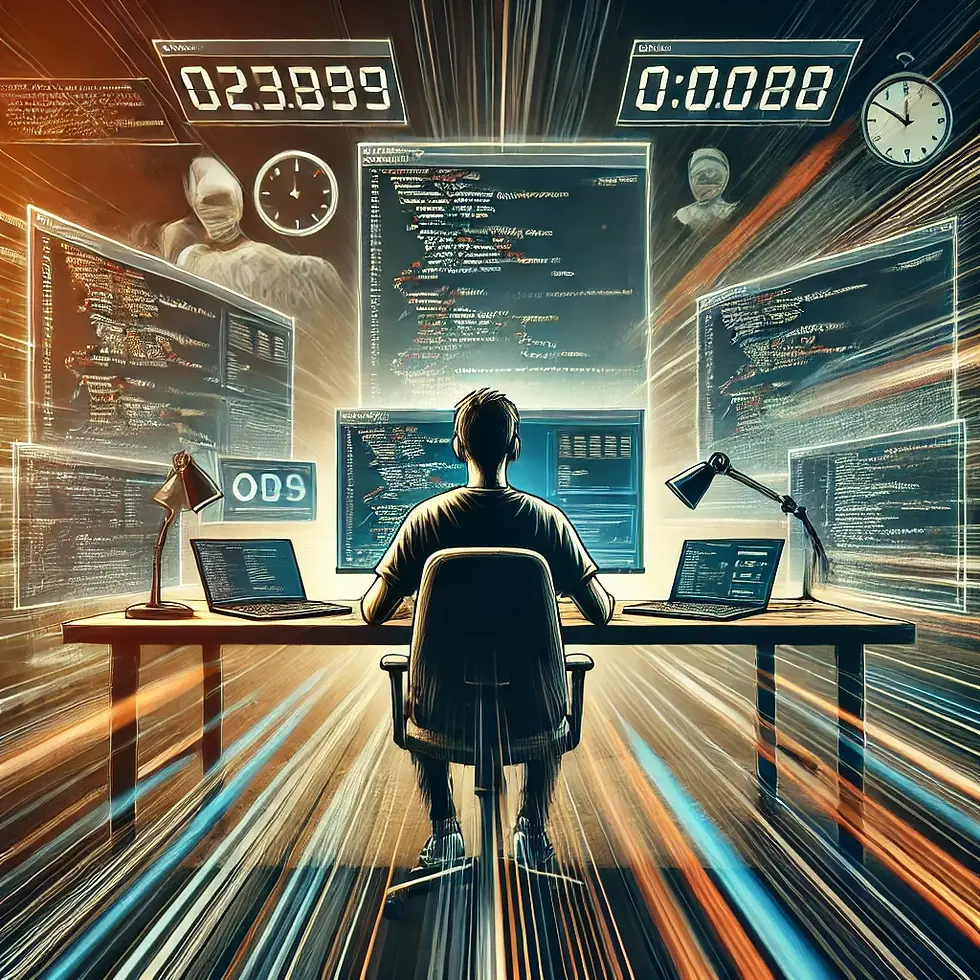
Introduction
In the fast-paced world of coding competitions and technical interviews, speed is crucial. With companies expecting swift yet accurate solutions, mastering efficiency can be a game-changer. Platforms like LeetCode, known for their time-constrained environments, are the perfect training grounds for improving your problem-solving speed.
This guide will help tech interview candidates, coding enthusiasts, and students boost their competitive programming skills with actionable tips and techniques.
Setting Up Your Workspace for Leetcode Speedruns
Ideal Editor/IDE Configurations
The right tools can make all the difference. Here's how to set up your workspace:
Choose a Fast IDE: VSCode, PyCharm, or Sublime Text are popular choices due to their speed and flexibility.
Enable Auto-Completion: This feature predicts code snippets, saving you typing time.
Customize Shortcuts: Set up keyboard shortcuts for frequently used commands (e.g., copy, paste, indent, run).
Essential Keyboard Shortcuts for Faster Navigation
Keyboard shortcuts are your best friend during speedruns. Here are some essential ones to master:
Auto-completion (Ctrl + Space): Quickly complete functions or variables.
Go to Definition (F12): Jump to a function or variable definition.
Switch Tabs (Ctrl + Tab): Move swiftly between open files.
Format Code (Shift + Alt + F): Auto-indent your code for readability.
Mental Shortcuts and Patterns
Recognizing Common Problem Patterns
Understanding common patterns can significantly cut down your problem-solving time:
Sliding Window: Efficiently process a window of elements within an array.
Two Pointers: Solve problems involving pairs of elements in linear time.
Example:
Let's apply this to"Longest Substring Without Repeating Characters." Recognizing this pattern helps solve the problem more efficiently than a brute force approach.
Problem Statement: Given a string s, find the length of the longest substring without repeating characters.
def lengthOfLongestSubstring(s: str) -> int:
char_set = set()
left = 0
max_length = 0
for right in range(len(s)):
while s[right] in char_set:
char_set.remove(s[left])
left += 1
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
Explanation:
Sliding Window: We use two pointers (left and right) to represent a "window" of characters within the string. The right pointer moves to explore new characters, while the left pointer adjusts to remove duplicates.
Set: A set is used to store characters in the current window, ensuring that there are no repeating characters.
Optimization: Instead of recalculating the substring from scratch every time, we move the left pointer to shrink the window when duplicates are found.
Example usage:
s = "abcabcbb"
print(lengthOfLongestSubstring(s))
Output: 3 (for "abc")
Initial Window

After a duplicate is found

Final Window

Developing Problem-Solving Templates
Let's make a template for the problem we solved
def sliding_window_template(s: str) -> int:
# Initialize necessary variables
window_elements = set() # This could be a set, dict, or other
structure based on problem
left = 0 # Left pointer
result = 0 # Variable to store the result (could be max_length, count, etc.)
# Iterate over the string with the right pointer
for right in range(len(s)):
# while condition to shrink the window when it becomes invalid
while s[right] in window_elements: # Modify condition based on problem
window_elements.remove(s[left])
left += 1 # Move the left pointer to shrink the window
# Expand the window by adding the current element
window_elements.add(s[right])
# Update the result based on the current window size
result = max(result, right - left + 1) # Modify result
calculation based on problem
return result
Key Elements:
Window Initialization:
window_elements: Can be a set, dictionary, or other structure based on the problem requirements.
Two Pointers:
left: Tracks the start of the window.
right: Iterates over the string or array, expanding the window.
While Loop:
Shrinks the window when a certain condition is violated (e.g., duplicate characters).
Result Update:
In this case, the result stores the maximum length, but it can be modified to count occurrences or sum values.
Adaptation Examples:
Sum of Subarray: Instead of checking for repeating characters, you could modify the template to track subarray sums.
Maximum or Minimum in Subarrays: Use a different data structure (e.g., deque) for tracking max/min values in sliding windows.
Time Management Strategies
Prioritizing Solving Easier Problems First

Start with easier problems to build confidence and score points quickly:
Easy-Medium-Hard Strategy: Tackle problems in ascending difficulty.
Quick Wins: Solve simpler problems to accumulate points and gain momentum.
Breaking Down Complex Problems Quickly
Simplify complex problems by breaking them down into manageable steps:
Identify Key Components: Break the problem into smaller parts.
Pseudocode: Outline your solution in pseudocode before coding.
Actionable Tip:
For your next 5 problems, spend a few minutes writing pseudocode to ensure clear thinking before implementation.
Optimizing Problem-Solving Flow
Reducing Context Switching
Minimize distractions by using mental models to stay focused:
Single-Tasking: Focus on one problem at a time.
Mental Models: Leverage familiar problem-solving models to streamline your approach.
Example:
Use pseudocode to outline your solution and reduce context switching. This practice keeps your mind focused on the problem at hand.
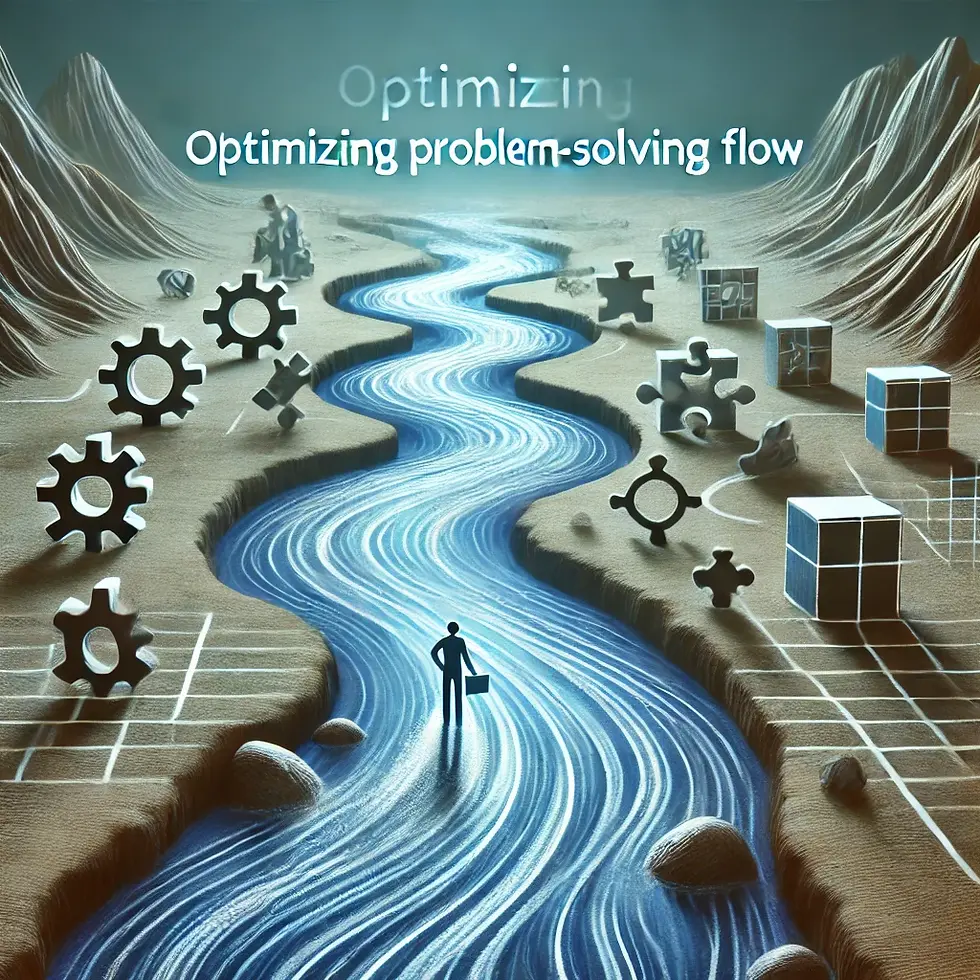
Using Templates for Common Problems
Pre-built templates can save time during contests:
Function Templates: Create reusable templates for common algorithms.
Quick Adaptation: Modify templates to fit specific problems quickly.
Practice Drills for Speed
Importance of Mock Speedrun Sessions
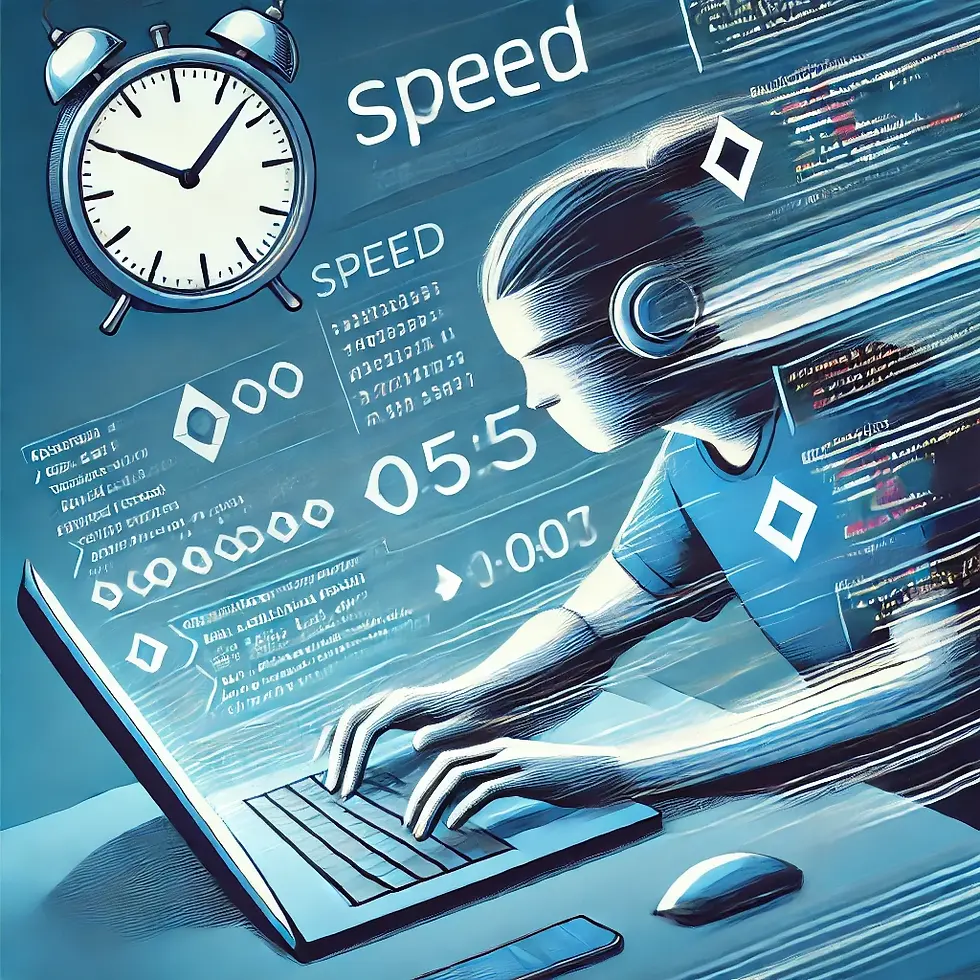
Simulate coding competitions to build stamina and improve speed:
Timed Practice: Solve multiple LeetCode problems within a set time frame.
Evaluate Performance: Review your performance and identify areas for improvement.
Actionable Tip:
Set aside 1 hour and attempt to solve 3-5 problems to build your problem-solving stamina.
Analyzing Your Mistakes
Review mistakes after every speedrun to refine your approach:
Track Mistakes: Keep a journal of mistakes and improvements.
Learn and Adapt: Use each mistake as a learning opportunity.
Actionable Tip:
Keep a journal of mistakes and improvements to track your progress.
Conclusion
Consistency is key in mastering efficiency in coding. By implementing these tips and regularly practicing, you can significantly improve your speed while maintaining accuracy. Remember, the goal is not just to solve problems quickly but to solve them effectively.
Take the first step towards becoming a faster, more efficient coder today. Implement these strategies in your next coding session and watch your skills soar!
Start your LeetCode speedrun practice now and see the difference! Happy coding!
Comments