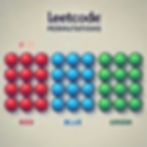
The permutations problem on Leetcode is a classic interview question that tests your understanding of recursion and backtracking. In this blog post, we will break down the problem, discuss the concepts involved, and provide a step-by-step guide to solving it with Python. This is leetcode permutation problem guide.
Understanding the Problem
The problem statement is as follows:
Given an array of distinct integers, return all possible permutations.
Input: nums = [1, 2, 3]
Output: [[1, 2, 3], [1, 3, 2], [2, 1, 3], [2, 3, 1], [3, 1, 2], [3, 2, 1]]
Key Concepts
To solve this problem, we need to understand the following concepts:
Recursion: A method of solving problems where a function calls itself.
Backtracking: A refinement of recursion where we build up a solution incrementally and remove solutions that fail to satisfy the problem constraints.
Backtracking Approach: Top-Down vs. Bottom-Up
In the context of solving the permutations problem, we use a top-down backtracking approach. This method involves fixing an element at the current position and recursively generating permutations for the remaining positions. This is in contrast to a bottom-up approach where you build the solution from smaller sub-problems.
Step-by-Step Solution
Step 1: Define the Function
First, we need to define our function permute which will take a list of numbers as input.
def permute(nums):
result = []
backtrack(nums, [], result)
return result
backtrack is the helper function which will be responsible for generating the permutations. It will take three arguments:
nums: The input list.
path: The current permutation being built.
result: The list of all permutations generated.
Using a helper function in the solution for the permutations problem is a common technique in recursive algorithms, especially when backtracking is involved. Here are the reasons why we use a helper function in this context:
Isolation of Logic: The helper function isolates the backtracking logic from the main function. This makes the code cleaner and more modular. By separating concerns, the main function (permute) only needs to handle the initialization and final result, while the helper function (backtrack) manages the recursive generation of permutations.
State Preservation: Using a helper function helps preserve the state of the current permutation (path) and the remaining elements (nums) across recursive calls. Each recursive call works with its own copy of the state, avoiding unintended side effects on other parts of the algorithm.
Step 2: Implement the Backtracking Function
def permute(nums):
results = []
backtrack(0, len(nums))
return results
def backtrack(start, end):
# Define a helper function for backtracking through the permutations.
# start: The starting index for generating permutations.
# end: The length of the list 'nums'.
if start == end:
# If the starting index equals the length of nums,
# we have a complete permutation.
# Append a copy of the current permutation to the results list.
results.append(nums[:])
for number in range(start, end):
# Iterate through the array from the current starting index to the end.
# Swap the element at the current index 'number' with the element at the starting index 'start'.
# This places the element at 'number' in the current position for this permutation.
nums[number], nums[start] = nums[start], nums[number]
# At this point, we have fixed the element at 'number' in position 'start'.
# Now, we recursively generate permutations for the remaining positions.
backtrack(start + 1, end)
# Backtrack by swapping the elements back to their original positions.
# This step is crucial to restore the array before the next iteration.
nums[number], nums[start] = nums[start], nums[number]
# Initialize the results list to store all the permutations.
results = []
# Call the backtrack function starting from the first index (0) to the length of the list.
backtrack(0, len(nums))
# Return the list of all generated permutations.
return results
Base Case: If the current permutation is of the same length as the original array, add it to the result list.
Recursive Case: Iterate through the array and construct permutations by appending each element to the current permutation, ensuring that each element is only used once per permutation.
Backtracking: Remove the last added element and try the next possibility.
Appending nums[:] Reason: Avoiding Reference Issues
A lot of people might wonder that while appending why we are doing
result.appending(nums[:])
instead of directly adding nums. The reason is that nums which is a list is a mutable object. When you append nums directly to results, you are appending a reference to the list nums. Because nums is a mutable object, any subsequent changes to nums will be reflected in all references to it, including those stored in results.
This means that if you change nums after appending it to results, the changes will appear in all the previously appended references, leading to incorrect results.
By appending a copy of nums (nums[:]), you ensure that the current state of nums at that particular recursive call is saved. Any subsequent changes to nums will not affect the stored permutations in results.
Main Logic Of Leetcode Permutation Problem Guide
The backtrack function starts by checking if the starting index (start) is equal to the end index (end). If this condition is met, it means we have generated a complete permutation, and we append a copy of this permutation to the results list. This base case ensures that each valid permutation is captured and stored.
Next, we iterate through the array from the current starting index to the end. During each iteration, we swap the element at the current index (number) with the element at the starting index (start). This swapping places the element at number in the current position for this permutation, effectively fixing it for this step of the recursion.
After fixing an element in position, we recursively call backtrack with the next starting index (start + 1). This recursive call generates permutations for the remaining positions in the list. Once the recursive call completes, we perform a swap to backtrack, restoring the original order of the elements before the next iteration.
This step is crucial as it ensures that the previous fixed element is unfixed, allowing us to fix a new element in the next iteration of the loop.
In the visualization, we use blanks (represented by __) to demonstrate how each element is chosen and placed in the permutation step by step. This method visually simplifies the concept by showing the process of filling in each position.
However, the actual implementation of the algorithm uses swapping. Swapping elements is an efficient way to generate permutations because it directly modifies the list in place, reducing the need for additional space. Each swap ensures that the current element is placed in the correct position, and subsequent recursive calls handle the rest of the list.
The choice-based visualization and the swap-based algorithm both achieve the same goal of generating permutations. The visualization helps in understanding the concept intuitively, while the swapping technique is a practical approach for implementation. Remember, the essence of both methods is to explore all possible arrangements of the elements, ensuring every permutation is generated correctly.
Follow-Up Questions
Permutations with Restrictions:
Question: How would you generate permutations of an array with certain elements fixed in specific positions?
Hint: Adjust the recursive backtracking function to skip fixed positions and only permute the remaining elements.
Permutations in a Matrix:
Question: Given an NxN matrix, how can you generate permutations of matrix rows such that no two rows are the same permutation?
Hint: Treat each row as an element and generate permutations while ensuring uniqueness among rows.
Permutation Game:
Question: Imagine a game where two players take turns choosing elements from a permutation. How can you determine if the first player can always win given optimal play?
Hint: Use game theory and recursive backtracking to analyze all possible game states and outcomes.
Conclusion
Understanding permutations and how to generate them is a fundamental skill in computer science, particularly in combinatorics and algorithm design. Through this blog post, we explored the concept of permutations, delved into a common permutation problem from LeetCode, and implemented a solution using Python's backtracking algorithm.
By visualizing the algorithm with both blanks and swaps, we clarified how permutations are constructed step-by-step, ensuring a comprehensive grasp of the process. Whether you're preparing for coding interviews or enhancing your algorithmic knowledge, mastering permutations will undoubtedly be a valuable addition to your skill set.
Keep experimenting with different problems and visualizations to deepen your understanding and improve your problem-solving abilities. Happy coding!